What Is an Expression?
An expression is a piece of code that produces a value. Think of it like a little math problem that JavaScript solves for you. When you write an expression, JavaScript evaluates it and gives you an answer. For example:
5 + 3
This expression uses the addition operator (+) to combine the numbers 5 and 3. When evaluated, it gives the value 8.
Arithmetic Operators
Arithmetic operators let you perform basic math calculations. The most common ones are:
- Addition (+): Combines two numbers.
Example: 5 + 3 gives 8. - Subtraction (-): Finds the difference between two numbers.
Example: 10 - 4 gives 6. - Multiplication (*): Multiplies two numbers.
Example: 4 * 2 gives 8. - Division (/): Divides one number by another.
Example: 20 / 5 gives 4. - Remainder (%): Gives the remainder after division.
Example: 7 % 3 gives 1.
Combining Operators in Expressions
You can combine values and operators to build more complex expressions. For instance, if you write:
(10 + 5) * 2
JavaScript first calculates the expression in parentheses (10 + 5 equals 15), and then multiplies that result by 2, giving 30.
Using Expressions with Variables
Often, you’ll use expressions with variables to update values. For example:
let counter = 0;
counter = counter + 1;
console.log(counter);
Here, the expression counter + 1 adds 1 to the current value of counter, and then we update counter with the new value.
Exercises
Simple Addition
- Write an expression that adds 5 and 3 together
- Store the result in a variable called result using let.
- Print result using console.log(result).
Combining Multiple Operators
- Create an expression that calculates the result of (8 * 2) - (10 / 2).
- Store the answer in a variable called calculation using let.
- Print the value of calculation with console.log(calculation).
Updating a Counter
- Create a variable called counter using let and initialize it to 0.
- Update the variable by adding 1 to it using an expression.
- Print the updated value using console.log(counter).
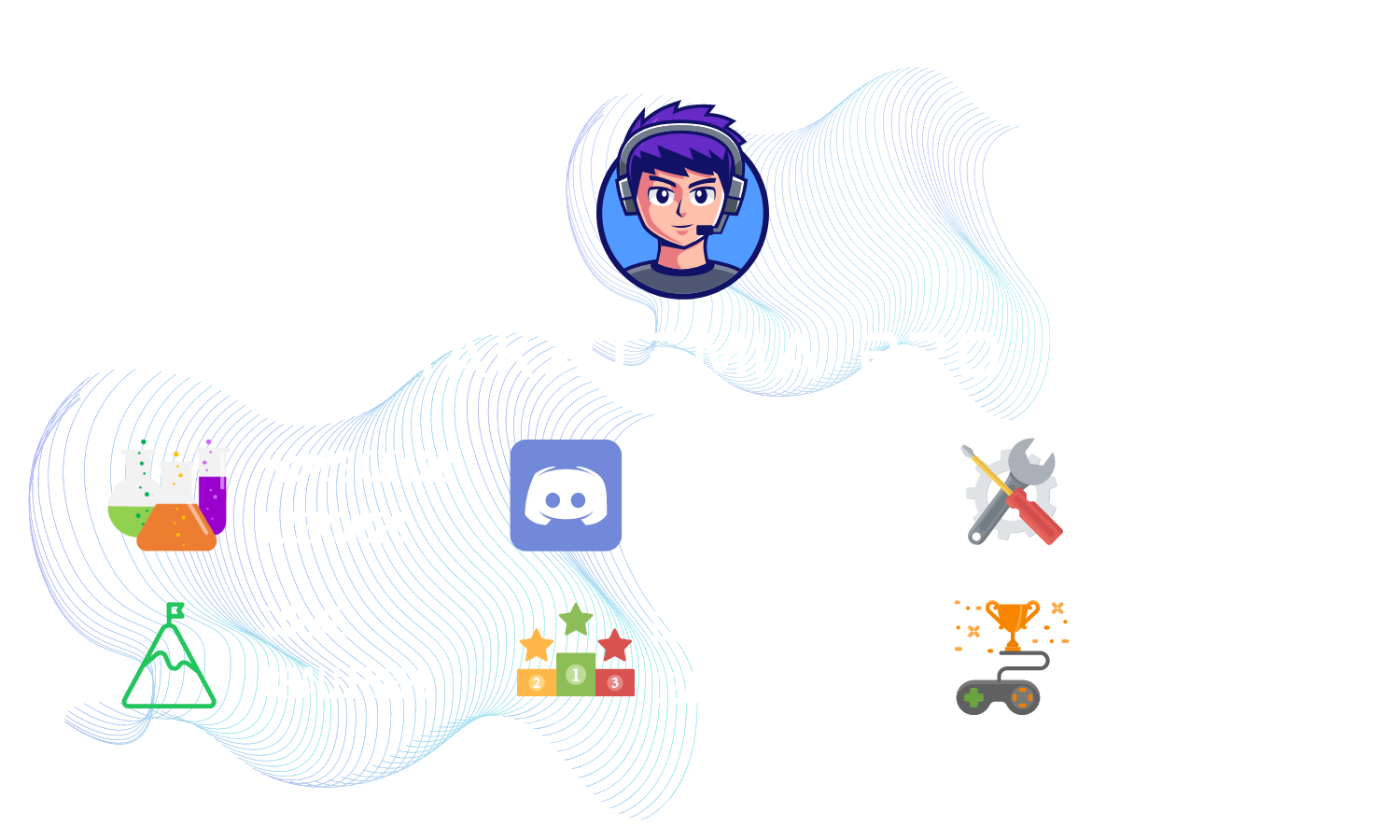
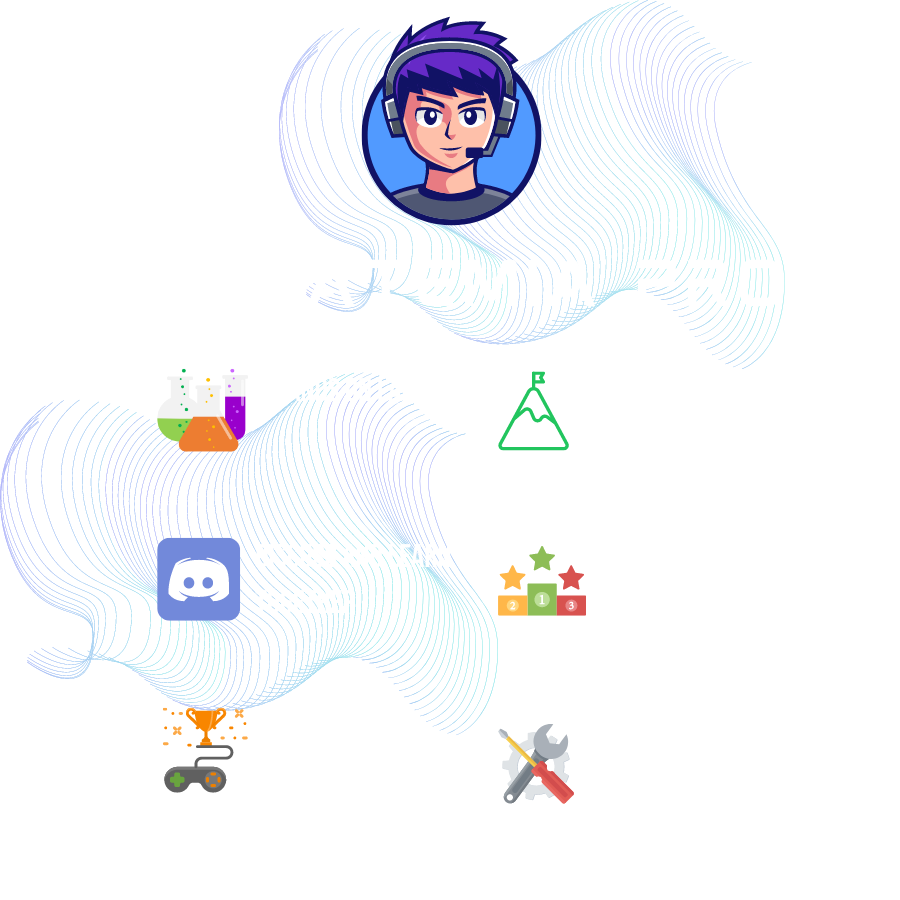
Ready to become an ethical hacker?
Start today.
As a member of Hakatemia you get unlimited access to Hakatemia modules, exercises and tools, and you get access to the Hakatemia Discord channel where you can ask for help from both instructors and other Hakatemia members.